Visual Studio Regular Expression
In visual basic, regular expression (regex) is a pattern and it is useful to parse and validate whether the given input text is matching the defined pattern (such as an email address) or not.
This extension provides tools for Visual Studio 2017 and 2019 that help you to create regular expressions. Regex Editor allows you to create and to test various aspects of the regular expressions Quick Ref Pane allows you to keep information on various elements of. In visual basic, regular expression (regex) is a pattern and it is useful to parse and validate whether the given input text is matching the defined pattern (such as an email address) or not. Generally, the key part to process the text with regular expressions is regular expression engine and it is represented by Regex class in visual basic. The Regex class is available with System.Text.
Generally, the key part to process the text with regular expressions is regular expression engine and it is represented by Regex
class in visual basic. The Regex
class is available with System.Text.RegularExpressions namespace.
To validate the given input text using regular expressions, the Regex
class will expect the following two items of information.
- The regular expression pattern to identity in the text.
- The input text to parse for the regular expression pattern.
Visual Basic Regular Expression Example
Following is the example of validating whether the given text is in proper email format or not using Regex
class in visual basic.
Imports System.Text.RegularExpressions
Module Module1
Sub Main(ByVal args AsString())
Dim email AsString = 'support@tutlane.com'
Dim result = Regex.IsMatch(email, '^[w-.]+@([w-]+.)+[w-]{2,4}$')
Console.Write('Is valid: {0} ', result)
Sims 4 all dlc free download mac. Console.ReadLine()
EndSub
EndModule
If you observe the above example, we are validating whether the input string (email) is in valid email format or not using Regex
class IsMatch
method by sending input string and the regular expression pattern to validate the input text.
When we execute the above code, we will get the result like as shown below.
This is how the Regex
class is useful to validate the input string by sending regular expression patterns based on our requirements.
Visual Basic Regex Class Methods
In visual basic, the Regex class is having a different methods to perform various operations on input string. The following table lists various methods of Regex class in c#.
Method | Description |
---|---|
IsMatch | It will determine whether the given input string matching with regular expression pattern or not. |
Matches | It will return one or more occurrences of text that matches the regular expression pattern. |
Replace | It will replace the text that matches the regular expression pattern. |
Split | It will split the string into an array of substrings at the positions that match the regular expression pattern. |
These Regex class methods are useful to validate, replace or split the string values by using regular expression patterns based on our requirements.
Visual Basic Regex Replace String Example
Following is the example of finding the substrings using regular expression patterns and replace with required values in visual basic.
Imports System.Text.RegularExpressions
Module Module1
Sub Main(ByVal args AsString())
Dim str AsString = 'Hi,welcome@to-tut#lane.com'
Dim result AsString = Regex.Replace(str, '[^a-zA-Z0-9_]+', ' ')
Console.Write('{0} ', result)
Console.ReadLine()
EndSub
EndModule
If you observe the above example, we used Regx.Replace method to find and replace all the special characters in a string with space using regular expression patterns ('[^a-zA-Z0-9_]+').
Here, the regular expression pattern ('[^a-zA-Z0-9_]+') will try to match any single character that is not in the defined character group.
When we execute the above example, we will get the result as shown below.
Visual Basic Regex Find Duplicate Words Example
Generally, while writing the content we will do common mistakes like duplicating the words. By using a regular expression pattern, we can easily identify duplicate words.
Following is the example of identifying the duplicate words in a given string using Regex class methods in visual basic.
Imports System.Text.RegularExpressions
Module Module1
Sub Main(ByVal args AsString())
Dim str AsString = 'Welcome To to Tutlane.com. Learn c# in in easily'
Dim collection AsMatchCollection = Regex.Matches(str, 'b(w+?)s1b', RegexOptions.IgnoreCase)
Visual Studio Regular Expression Replace
ForEach m AsMatchIn collection
Fiddler mac os x download. Console.WriteLine('{0} (duplicates '{1}') at position {2}', m.Value, m.Groups(1).Value, m.Index)
Next
Console.ReadLine()
EndSub
EndModule
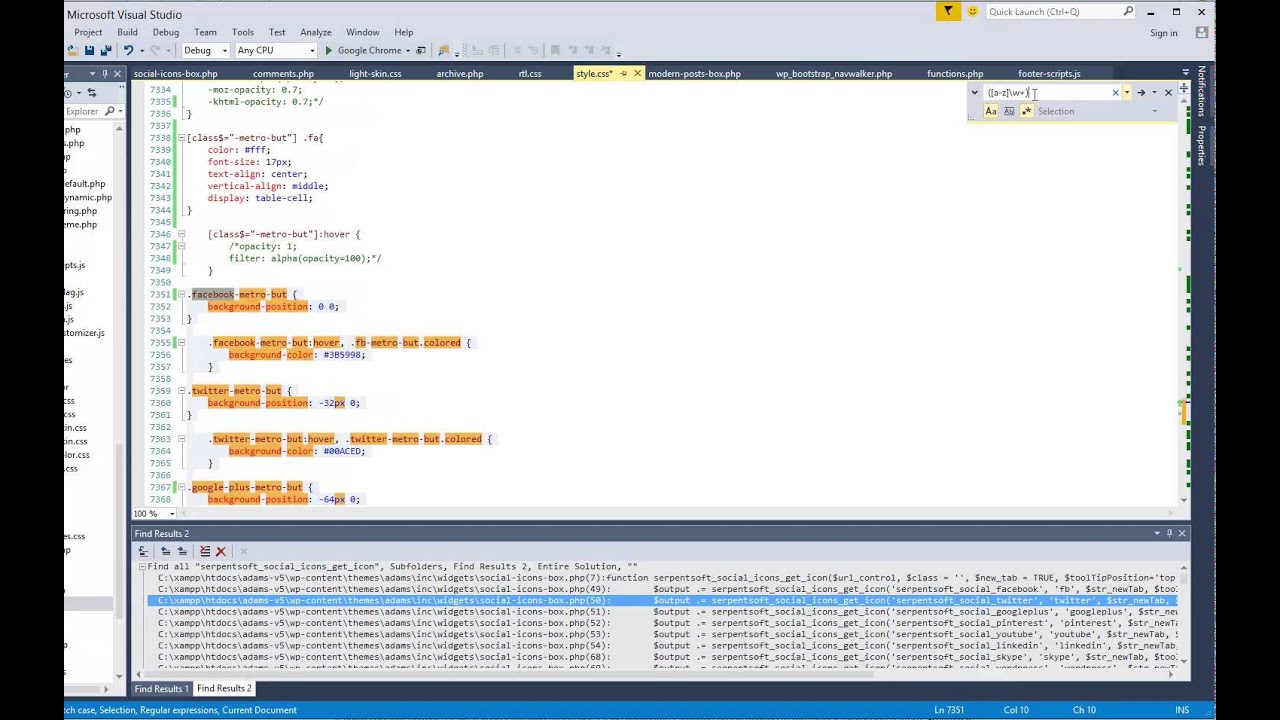
If you observe the above example, we used Regx.Matches method to find the duplicated words using regular expression pattern ('b(w+?)s1b').
Here, the regular expression pattern ('b(w+?)s1b') will perform a case-insensitive search and identify the duplicate words which exist side by side like (Toto or in in).
When we execute the above example, we will get the result as shown below.
To to (duplicates 'To') at position 8
in in (duplicates 'in') at position 36
This is how we can use regular expressions in visual basic to parse and validate the given string based on our requirements.
Translator: Crazy Technology House
https://medium.freecodecamp.o…
Wechat Public Number: Front-end Pioneer
Welcome to pay attention and push you fresh front-end technology articles every day
Do you always want to learn regular expressions, but it’s delayed because of its complexity? In this article, I will show you five easy to learnregularSkills, you can use them immediately in your favorite text editor.
Text Editor Settings
Although almost all text editors now support regular expressions, I use Visual Studio Code in this tutorial, but you can use any editor you like. Also note that you usually need to turn on the RegEx switch somewhere near the search input box. The following is how to do this in VS Code:
You need to enable RegEx by selecting this option
1) .
Match any character
Let’s get started. Dot symbol.
Used to match any character:
The above regular matching'bot'
,`'bat'
And anyb
At the beginning,t
A word with three characters at the end. But if you want to search for dot symbols, you need to useTo escape it, so the following rule only matches the exact text
'b.t'
:
2) .*
Match anything
Here.
Express“Any character”, *
Express“This symbol repeats the previous content any number of times.”Put them together(.*
) Representation“Repeat any symbol any number of times.”For example, you can use it to find matches that begin or end with some text. Suppose we have a JavaScript method like this:
We want to find all calls to this method, wherepathToFile
Point to folders“lua”
Any file in the file. The following regular expressions can be used:
That means,“Match all with'loadScript'
Start with'lua'
The end of the string.”
3) ?
Non-greedy Matching
.*
Later?
Symbols and other matching rules mean “matching as little as possible”. In the previous image, each match was made twice.'lua'
String, until the second'lua'
All things match up. If you want to match the first one'lua'
The following rules can be used:
That means,“Match all with'loadScript'
The beginning, followed by any character, until the first appearance'lua'
loadScript.*?lua
Match everything that starts with loadScript until the first “lua” appears
4) `() capture group and reverse reference
Okay, now we can match some words. But what if you want to modify some of the text we find? The capture group is then used.
Suppose we modify it.loadScript
Method, now you need to insert another parameter between its original two parameters. Let’s name this new parameterid
At this time, the new function prototype should be as follows:loadScript(scriptName,id,pathToFile)
。 We can’t replace the normal functionality of a text editor here, but regular expressions can help us.
You can see the results of running the following regular expressions from the figure above.
This means:“Match to'loadScript('
At the beginning, follow any content until you meet the first one.,
And then anything, until the first)
”
Perhaps the only thing that seems strange to you isSymbol. They are used to escape parentheses.
Because symbols(
and)
Regular expressions are used to capture special characters that match part of the text, but we need to match actual parentheses, so we need to escape them.
In the previous expression, we used.*?
Symbols define two parameters of a method call. Make each parameter separateCapture groupYou need to add them before and after(
and)
Symbol:
If you run this rule, you will see no change. This is because it matches the same text. But now we can call the first parameter$1
The second parameter is called$2
。 This is called a reverse reference, which will help us do what we want: add another parameter between two parameters:
Search input:
Lego batman 2 free download mac. This is the same as the previous regularization, but the parameters are mapped to inverted capture groups 1 and 2, respectively.
Replace input:
That means“Text'loadScript('
Capture group 1,'id'
Capture group 2 and)
Replace each matching text. Note that you do not need to replace input escape brackets.
5) [ ]
Character class
You can be at [
and]
List the characters to match in a particular location within the symbol. For example,[0-9]
Match all numbers from 0 to 9. You can also clearly list all the numbers:[0123456789]
—— The meaning is the same as before. You can also use alphabetic dashes.[a-z]
All lowercase Latin characters will be matched.[A-Z]
All uppercase Latin characters will be matched.[a-zA-Z]
It will match both.
You can also use it after the character class*
Like in.
Later, as in this case, it means:“Match any number of characters in this class”
Epilogue
You should know how to write regular expressions. I’m talking about the JavaScript RegEx engine here. Most modern engines are similar, but there may be some differences. Usually these differences include escape characters and reverse reference tags.
You can now open the text editor and start using some of these techniques immediately. You will see that many refactoring tasks can be accomplished faster than before. Once you have mastered these techniques, you can begin to study more regular expressions.
Visual Studio Regular Expressions Multiline
Wechat Public Number: Front-end Pioneer
Welcome to scan the two-dimensional code, pay attention to the public number, and push you fresh front-end technical articles every day.
Welcome to continue reading other highly praised articles in this column:
Visual Studio Regular Expression New Line
- Twelve amazing CSS pilot projects
- Fifty React Interview Questions Must Be Meet
- What do top companies in the world ask in front-end interviews?
- 11 Best JavaScript Dynamic Effect Libraries
- CSS Flexbox Visualization Manual
- React from the Designer’s Perspective
- Is the festival boring? Write a brain game in JavaScript.
- How does CSS sticky positioning work
- Step by step teach you how to use HTML5 SVG to achieve animation effect
- Programmers earn less than 30K a month before they are 30 years old. Where to go?
- 14 Best JavaScript Data Visualization Libraries
- Eight top-level VS Code extension plug-ins for the front end
- Complete guide to Node.js multithreading
- Four Solutions and Implementation of Converting HTML to PDF
